概述
本文用AndroidStudio制作一个非常简单的计算器app。目的是熟悉前端设计元素和后端代码执行逻辑,以及二者的整合。
演示
能实现最简单的四则运算:
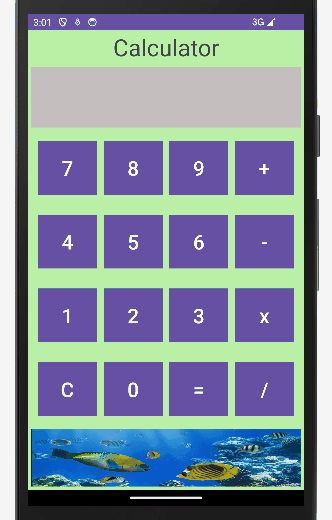
代码
前端:activity_main.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223
| <?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity" android:background="@color/whole_bg">
<LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical">
<TextView android:layout_width="match_parent" android:layout_height="50dp" android:text="Calculator" android:textSize="35dp" android:gravity="center"></TextView>
<TextView android:id="@+id/tv_display" android:layout_width="match_parent" android:layout_height="90dp" android:text="" android:textSize="60dp" android:background="#C5BEBE" android:layout_margin="5dp" android:gravity="right" ></TextView>
<LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="vertical">
<LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:weightSum="4" android:padding="10dp"> <Button android:id="@+id/btn_7" android:text="7" android:textSize="30dp" android:layout_width="0dp" android:layout_weight="1" android:layout_height="80dp" android:background="@color/button_bg" android:layout_margin="5dp"></Button> <Button android:id="@+id/btn_8" android:text="8" android:textSize="30dp" android:layout_width="0dp" android:layout_weight="1" android:layout_height="80dp" android:background="@color/button_bg" android:layout_margin="5dp"></Button> <Button android:id="@+id/btn_9" android:text="9" android:textSize="30dp" android:layout_width="0dp" android:layout_weight="1" android:layout_height="80dp" android:background="@color/button_bg" android:layout_margin="5dp"></Button> <Button android:id="@+id/btn_add" android:text="+" android:textSize="30dp" android:layout_width="0dp" android:layout_weight="1" android:layout_height="80dp" android:background="@color/operator_bg" android:layout_margin="5dp"></Button> </LinearLayout>
<LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:weightSum="4" android:padding="10dp"> <Button android:id="@+id/btn_4" android:text="4" android:textSize="30dp" android:layout_width="0dp" android:layout_weight="1" android:layout_height="80dp" android:background="@color/button_bg" android:layout_margin="5dp"></Button> <Button android:id="@+id/btn_5" android:text="5" android:textSize="30dp" android:layout_width="0dp" android:layout_weight="1" android:layout_height="80dp" android:background="@color/button_bg" android:layout_margin="5dp"></Button> <Button android:id="@+id/btn_6" android:text="6" android:textSize="30dp" android:layout_width="0dp" android:layout_weight="1" android:layout_height="80dp" android:background="@color/button_bg" android:layout_margin="5dp"></Button> <Button android:id="@+id/btn_minus" android:text="-" android:textSize="30dp" android:layout_width="0dp" android:layout_weight="1" android:layout_height="80dp" android:background="@color/operator_bg" android:layout_margin="5dp"></Button> </LinearLayout>
<LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:weightSum="4" android:padding="10dp"> <Button android:id="@+id/btn_1" android:text="1" android:textSize="30dp" android:layout_width="0dp" android:layout_weight="1" android:layout_height="80dp" android:background="@color/button_bg" android:layout_margin="5dp"></Button> <Button android:id="@+id/btn_2" android:text="2" android:textSize="30dp" android:layout_width="0dp" android:layout_weight="1" android:layout_height="80dp" android:background="@color/button_bg" android:layout_margin="5dp"></Button> <Button android:id="@+id/btn_3" android:text="3" android:textSize="30dp" android:layout_width="0dp" android:layout_weight="1" android:layout_height="80dp" android:background="@color/button_bg" android:layout_margin="5dp"></Button> <Button android:id="@+id/btn_multiple" android:text="x" android:textSize="30dp" android:layout_width="0dp" android:layout_weight="1" android:layout_height="80dp" android:background="@color/operator_bg" android:layout_margin="5dp"></Button> </LinearLayout>
<LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:weightSum="4" android:padding="10dp"> <Button android:id="@+id/btn_ac" android:text="C" android:textSize="30dp" android:layout_width="0dp" android:layout_weight="1" android:layout_height="80dp" android:background="@color/button_bg" android:layout_margin="5dp"></Button> <Button android:id="@+id/btn_0" android:text="0" android:textSize="30dp" android:layout_width="0dp" android:layout_weight="1" android:layout_height="80dp" android:background="@color/button_bg" android:layout_margin="5dp"></Button> <Button android:id="@+id/btn_cal" android:text="=" android:textSize="30dp" android:layout_width="0dp" android:layout_weight="1" android:layout_height="80dp" android:background="@color/button_bg" android:layout_margin="5dp"></Button> <Button android:id="@+id/btn_divide" android:text="/" android:textSize="30dp" android:layout_width="0dp" android:layout_weight="1" android:layout_height="80dp" android:background="@color/operator_bg" android:layout_margin="5dp"></Button> </LinearLayout>
</LinearLayout>
<ImageView android:id="@+id/iv_ad" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@drawable/fish" android:layout_margin="5dp" ></ImageView>
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
|
后端:MainActivity.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175
| package com.example.myproject02;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.TextView; import android.widget.Toast;
public class MainActivity extends AppCompatActivity { TextView tv_display; Button btn_0,btn_1,btn_2,btn_3,btn_4,btn_5,btn_6,btn_7,btn_8,btn_9; Button btn_add,btn_minus,btn_multiple,btn_divide; Button btn_ac, btn_cal; float f_temp1, f_temp2, res; String operator;
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main);
f_temp1 = 0.0f; f_temp2 = 0.0f; res = 0.0f; operator = "";
tv_display = findViewById(R.id.tv_display); btn_0 = findViewById(R.id.btn_0); btn_1 = findViewById(R.id.btn_1); btn_2 = findViewById(R.id.btn_2); btn_3 = findViewById(R.id.btn_3); btn_4 = findViewById(R.id.btn_4); btn_5 = findViewById(R.id.btn_5); btn_6 = findViewById(R.id.btn_6); btn_7 = findViewById(R.id.btn_7); btn_8 = findViewById(R.id.btn_8); btn_9 = findViewById(R.id.btn_9); btn_add = findViewById(R.id.btn_add); btn_minus = findViewById(R.id.btn_minus); btn_multiple = findViewById(R.id.btn_multiple); btn_divide = findViewById(R.id.btn_divide); btn_ac = findViewById(R.id.btn_ac); btn_cal = findViewById(R.id.btn_cal);
ClickAddString click_addstr = new ClickAddString(); ClickAdd click_add = new ClickAdd(); ClickMinus click_minus = new ClickMinus(); ClickMultiple click_multip = new ClickMultiple(); ClickDivide click_divide = new ClickDivide(); ClickAc click_ac = new ClickAc(); ClickCal click_cal = new ClickCal(); btn_0.setOnClickListener(click_addstr); btn_1.setOnClickListener(click_addstr); btn_2.setOnClickListener(click_addstr); btn_3.setOnClickListener(click_addstr); btn_4.setOnClickListener(click_addstr); btn_5.setOnClickListener(click_addstr); btn_6.setOnClickListener(click_addstr); btn_7.setOnClickListener(click_addstr); btn_8.setOnClickListener(click_addstr); btn_9.setOnClickListener(click_addstr); btn_add.setOnClickListener(click_add); btn_minus.setOnClickListener(click_minus); btn_multiple.setOnClickListener(click_multip); btn_divide.setOnClickListener(click_divide); btn_ac.setOnClickListener(click_ac); btn_cal.setOnClickListener(click_cal); }
class ClickAddString implements View.OnClickListener { @Override public void onClick(View v) { String str = tv_display.getText().toString(); Button btn_this = (Button) v; str = str + ((Button) v).getText(); tv_display.setText(str); } }
class ClickAdd implements View.OnClickListener{ @Override public void onClick(View v) { String s1 = tv_display.getText().toString(); f_temp1 = Float.parseFloat(s1); operator = "+"; tv_display.setText(""); } }
class ClickMinus implements View.OnClickListener{ @Override public void onClick(View v) { String s1 = tv_display.getText().toString(); f_temp1 = Float.parseFloat(s1); operator = "-"; tv_display.setText(""); } }
class ClickMultiple implements View.OnClickListener{ @Override public void onClick(View v) { String s1 = tv_display.getText().toString(); f_temp1 = Float.parseFloat(s1); operator = "x"; tv_display.setText(""); } }
class ClickDivide implements View.OnClickListener{ @Override public void onClick(View v) { String s1 = tv_display.getText().toString(); f_temp1 = Float.parseFloat(s1); operator = "/"; tv_display.setText(""); } }
class ClickAc implements View.OnClickListener{ @Override public void onClick(View v) { tv_display.setText(""); f_temp1 = 0.0f; f_temp2 = 0.0f; operator = ""; } }
class ClickCal implements View.OnClickListener{ @Override public void onClick(View v) { String s2 = tv_display.getText().toString(); f_temp2 = Float.parseFloat(s2); float res = calculate(); tv_display.setText(Float.toString(res)); } }
float calculate(){ switch(operator){ case "+": res = f_temp1 + f_temp2; break; case "-": res = f_temp1 - f_temp2; break; case "x": res = f_temp1 * f_temp2; break; case "/": res = f_temp1 / f_temp2; break; default: res = -11111111.0f; Toast.makeText(MainActivity.this, "error", Toast.LENGTH_SHORT).show(); } return res; }
}
|
额外定义的颜色:values/colors.xml
1 2 3 4 5 6 7 8
| <?xml version="1.0" encoding="utf-8"?> <resources> <color name="black">#FF000000</color> <color name="white">#FFFFFFFF</color> <color name="whole_bg">#BAF1A6</color> <color name="button_bg">#DB2D9C</color> <color name="operator_bg">#FF5722</color> </resources>
|
AndroidManifest.xml
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| <?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools">
<application android:allowBackup="true" android:dataExtractionRules="@xml/data_extraction_rules" android:fullBackupContent="@xml/backup_rules" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/Theme.MyProject02" tools:targetApi="31"> <activity android:name=".MainActivity" android:exported="true"> <intent-filter> <action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application>
</manifest>
|