读取txt文件内容并打印
fopen()
利用fopen()函数读取txt文件并打印其中的每一个字符:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| #include <stdio.h> #include <stdlib.h> #include <string.h>
int main() { FILE* file = fopen("example.txt", "r"); if (file == NULL) { printf("无法打开文件。\n"); return 1; }
char buffer[100]; while (fgets(buffer, sizeof(buffer), file)) { char* token = strtok(buffer, " \t\n"); while (token != NULL) { printf("%s ", token); token = strtok(NULL, " \t\n"); } printf("\n"); }
fclose(file); return 0; }
|
txt文件内容:

代码运行后cmd输出:
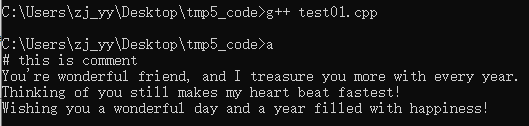
ifstream
利用ifstream和istringstream读取txt文件并打印其中的每一个字符:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| #include <iostream> #include <fstream> #include <sstream> #include <string>
int main() { std::ifstream file("example.txt"); if (!file) { std::cout << "无法打开文件。" << std::endl; return 1; }
std::string line; while (std::getline(file, line)) { std::istringstream iss(line); std::string word; while (iss >> word) { std::cout << word << " "; } std::cout << std::endl; }
file.close(); return 0; }
|
代码运行后cmd输出:
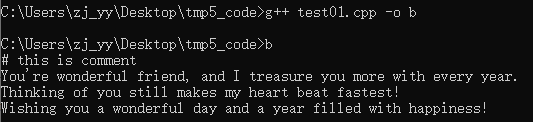